1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
System.out.print("값을 입력하라 : ");
int a = scan.nextInt();
while(a < 1) {
System.out.print("0이나 그 이하의 값은 불가능, 값 재입력 : ");
a = scan.nextInt();
}
for(int i = 1; i <= a; i++) {
for(int j = 1; j <= i; j++) {
if(j<i) {
System.out.print("@");
}else {
System.out.print("*");
}
}
System.out.println();
}
};
};
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
int a;
int n;
do {
System.out.print("출력 줄수 : ");
a = scan.nextInt();
}while(a <= 1);
System.out.println();
n = a*2-1;
for(int i = 1; i <= a; i++) {
for(int j = 1; j <= n; j++) {
if(j<i) {
System.out.print(" ");
}else {
System.out.print("*");
}
}
n--;
System.out.println();
}
};
};
|
cs |
역삼각형이 아닌 정삼각형을 만들고 싶을 때는 아래의 코드가 된다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
int a;
int n;
int s;
do {
System.out.print("출력 줄수 : ");
a = scan.nextInt();
}while(a <= 1);
s = n = a;
System.out.println();
for(int i = 1; i <= a; i++) {
for(int j = 1; j <= n; j++) {
if(j<s) {
System.out.print(" ");
}else {
System.out.print("*");
}
}
s--;
n++;
System.out.println();
}
};
};
|
cs |
또는
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
int a;
do {
System.out.print("출력 줄수 : ");
a = scan.nextInt();
}while(a <= 1);
System.out.println();
for(int i = 1; i <= a; i++) {
for(int j = 1; j <= i+a-1; j++) {
if(j<a+1-i) {
System.out.print(" ");
}else {
System.out.print("*");
}
}
System.out.println();
}
};
};
|
cs |
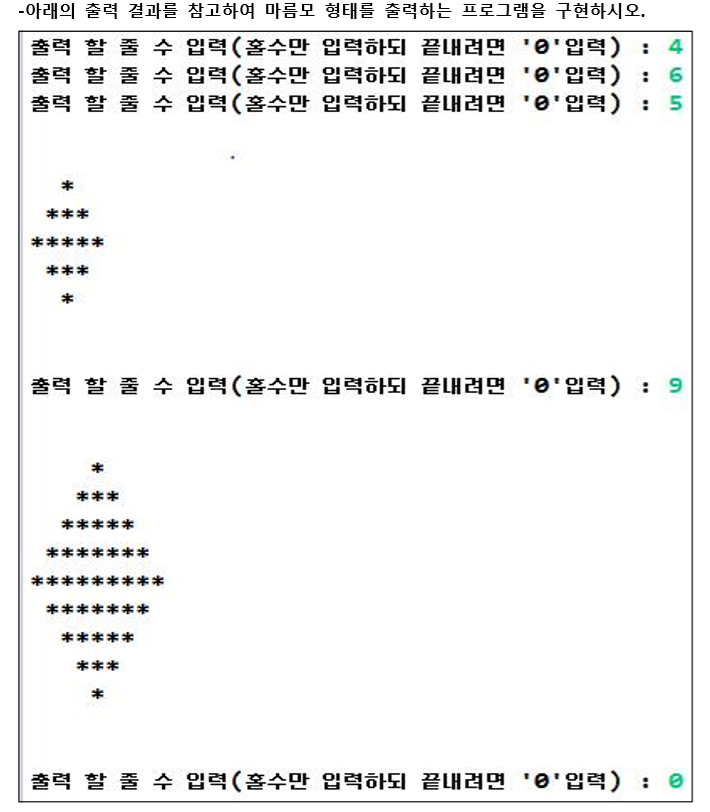
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
|
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
int a, b, c, d;
// do while 반복문으로 짝수면서 0이
// 아닐 때 재입력 할수 있게 만든 루프다.
do {
System.out.print("출력 할 줄 수 입력(종료하려면 '0'입력) : ");
a = scan.nextInt();
}while(a %2 == 0 && a != 0);
// if 조건문으로 0일때 종료되고 이외일 때 별찍기 실행
if(a == 0) {
System.out.print("");
}else {
b = (a+1)/2;
c = d = b;
System.out.println();
for(int i = 1; i <= a; i++) {
for(int j = 1; j <= d; j++) {
if(j < c) System.out.print(" ");
else System.out.print("*");
}
System.out.println();
if(i < b) {
c--;
d++;
}else {
c++;
d--;
}
}
};
};
};
|
cs |
'프로그래밍 공부 > Java' 카테고리의 다른 글
Java - 클래스(Class)와 객체(Object) 예제 (0) | 2020.02.05 |
---|---|
Java - 배열 (0) | 2020.02.03 |
Java - 반복문으로 별찍기 예제 (0) | 2020.01.23 |
Java - 반복문 (0) | 2020.01.21 |
Java - 조건문 (0) | 2020.01.21 |