이전에 GET, POST, PUT, DELETE 요청과 응답에 대해서 알아보았고, 이번에 알아볼 내용은 직접 데이터를 주고 받는 방법에 대한 내용이다. POST 요청으로 직접 데이터에 접근해 데이터 객체를 삽입하고 적용시키는 방법이기도 하다.
먼저 Node.js에 body-parser 미들웨어를 설치해야한다. 간단하게 터미널이나 명령 프롬프트에 npm install body-parer를 입력하면 설치 할 수 있다.
1
2
|
const bodyParser = require('body-parser')
app.use(bodyParser.urlencoded({ extended: false }))
|
cs |
자바스크립트 코드에 위의 코드를 추가해 주어야 한다. 코드를 추가하면 HTML 파일로 넘어가 POST 요청과 아래의 body 태그에 각각 값을 받을 .val() 메서드와 input 태그를 입력해준다.
1
2
3
4
5
6
7
|
<body>
<h1>품명 추가하기</h1>
<span>품명 : </span>
<input type="text" id="name"><br>
<span>가격 : </span>
<input type="text" id="price">
</body>
|
cs |
body 태그 내부의 input 태그로 값을 입력 받는다, 각각의 input 태그에는 아이디를 지정해 아이디를 받을 수 있도록 한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
<script>
$("#post-all").click(function () {
$.ajax({
url: "/drink",
method: "POST",
data: {
name: $("#name").val(),
price: $("#price").val()
},
dataType: "text",
success: function (data) {
$('#output').val(data)
console.log(data)
}
})
})
</script>
|
cs |
스트립트 안에 POST 요청부분에 data: { }를 추가하고, 아까 지정하였던 아이디를 받아 .val() 메서드를 이용해 값을 받을 수 있게 만들어준다.
아래는 완성된 HTML 코드이다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
|
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>express</title>
<script src="jquery-3.4.1.js"></script>
</head>
<body>
<h1>Hello World Static File</h1>
<button id="get-all">데이터 모두 가져오기</button>
<button id="post-all">데이터 추가하기</button>
<button id="get-one">0번 데이터 가져오기</button>
<button id="put-one">0번 데이터 수정하기</button>
<button id="delete-one">0번 데이터 제거하기</button>
<textarea name="" id="output" cols="40" rows="10"></textarea>
<script>
$("#get-all").click(function () {
$.ajax({
url: "/drink",
method: "GET",
data: {
a: "Hello_1",
b: "Hello_2"
},
dataType: "text",
success: function (data) {
$('#output').val(data)
console.log(data)
}
})
})
$("#post-all").click(function () {
$.ajax({
url: "/drink",
method: "POST",
data: {
name: $("#name").val(),
price: $("#price").val()
},
dataType: "text",
success: function (data) {
$('#output').val(data)
console.log(data)
}
})
})
$("#get-one").click(function () {
$.ajax({
url: "/drink/0",
method: "GET",
dataType: "text",
success: function (data) {
$('#output').val(data)
console.log(data)
}
})
})
$("#put-one").click(function () {
$.ajax({
url: "/drink/0",
method: "PUT",
dataType: "text",
success: function (data) {
$('#output').val(data)
console.log(data)
}
})
})
$("#delete-one").click(function () {
$.ajax({
url: "/drink/0",
method: "DELETE",
dataType: "text",
success: function (data) {
$('#output').val(data)
console.log(data)
}
})
})
</script>
<hr>
<h1>품명 추가하기</h1>
<span>품명 : </span>
<input type="text" id="name"><br>
<span>가격 : </span>
<input type="text" id="price">
</body>
</html>
|
cs |
그리고 완성된 자바스크립트 코드이다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
|
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
const items = [{
name: '우유',
price: '2000'
}, {
name: '홍차',
price: '5000'
}, {
name: '커피',
price: '5000'
}]
app.use(express.static('project'))
app.use(bodyParser.urlencoded({ extended: false }))
app.get("/drink", (request, response) => {
response.send(items)
})
app.post("/drink", (request, response) => {
console.log(request.body)
items.push({
name: request.body.name,
price: request.body.price
})
response.send(items[items.length - 1])
})
app.get("/drink/:id", (request, response) => {
const id = Number(request.params.id)
response.send(items[id])
})
app.put("/drink/:id", (request, response) => {
const id = Number(request.params.id)
items[id].name = "테스트"
items[id].price = 1000
response.send(items[id])
})
app.delete("/drink/:id", (request, response) => {
const id = Number(request.params.id)
items.splice(id, 1)
response.send("success")
})
app.listen(52273, () => {
console.log('Server Running at http://127.0.0.1:52273')
})
|
cs |
Node.js로 실행을 하고, input 입력란에 코코아와 3000을 각각입력후 데이터 추가하기를 누르면 명령 프롬프트(터미널)에 입력된 값을 받아온 것이 출력이되고, 데이터 모두 가져오기를 누르면 기존의 데이터에 {"name":"코코아","price":"4000"} 가 추가된 것을 알 수 있다.
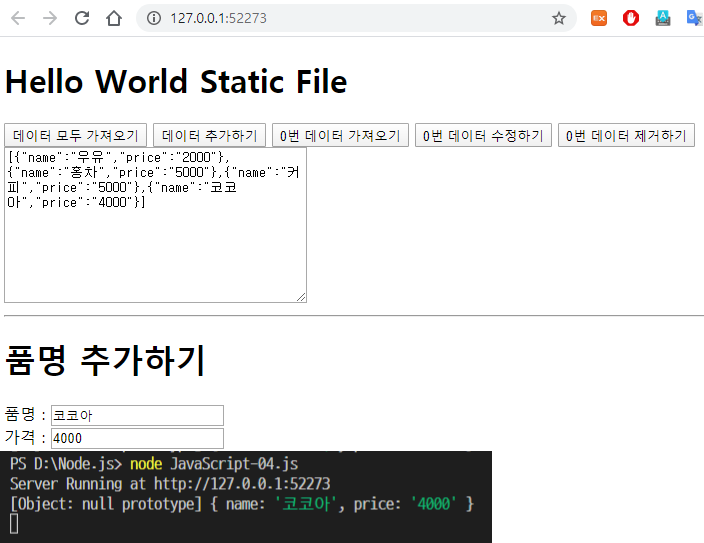
'프로그래밍 공부 > Ajax' 카테고리의 다른 글
Ajax - GET, POST, PUT, DELETE 요청과 응답 (0) | 2020.01.07 |
---|---|
Ajax - jQuery로 GET 요청하기 (0) | 2020.01.06 |
Ajax - express로 GET 요청 서버 만들기 (0) | 2020.01.03 |
Ajax - Ajax 개요 및 Node.js 기본 (0) | 2020.01.02 |